Hand Tracking Hinting¶
What is Hand Tracking Hinting?¶
Hand Tracking Hinting is the ability to change features of the hand tracking system based on specific needs of your application.
Available Hints are listed in the LeapC API documentation defined by "Request Using...".
e.g. “ultra_performance_mode”.
How do I apply Hand Tracking Hints on startup?¶
To apply Hand Tracking Hints on startup, you can populate a collection of text strings in the Ultraleap Settings panel.
Open the Ultraleap Settings panel
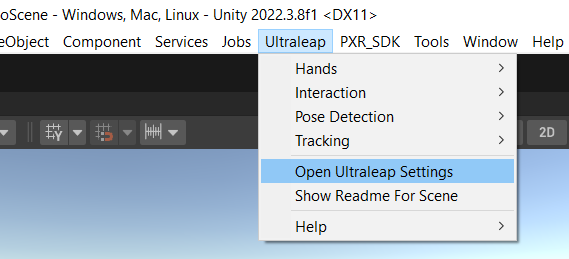
Populate the Startup Hints section with all hints you wish to enable on startup of your application
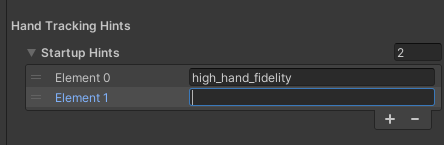
Run your application
How do I change Hand Tracking Hints at runtime?¶
You can request new collections of Hand Tracking Hints at runtime. We provide a HandTrackingHintManager class to do this.
Here is some sample code to explore what options you have at runtime:
using Leap;
using UnityEngine;
public class HintTest : MonoBehaviour
{
void Update()
{
if(Input.GetKeyDown(KeyCode.S)) // set the index finger pointing hint
{
HandTrackingHintManager.RequestHandTrackingHints(
new string[]
{
"straight_index_fingers",
"high_hand_fidelity"
}
);
}
if (Input.GetKeyDown(KeyCode.A)) // add to the existing hints
{
HandTrackingHintManager.AddHint("straight_index_fingers");
}
if (Input.GetKeyDown(KeyCode.R)) // remove from the existing hints
{
HandTrackingHintManager.RemoveHint("straight_index_fingers");
}
if (Input.GetKeyDown(KeyCode.C)) // clear the existing hints
{
HandTrackingHintManager.RequestHandTrackingHints(new string[] { });
}
}
}